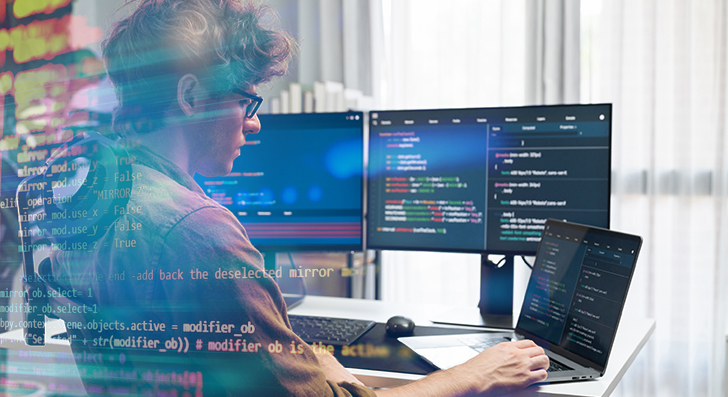
Scalability suggests your software can cope with progress—more buyers, far more info, and even more visitors—without breaking. To be a developer, constructing with scalability in mind saves time and worry later on. Here’s a transparent and useful guide to assist you to start off by Gustavo Woltmann.
Style for Scalability from the beginning
Scalability just isn't some thing you bolt on afterwards—it should be section of the approach from the beginning. Lots of programs fail every time they expand speedy due to the fact the first design and style can’t tackle the extra load. For a developer, you should Believe early regarding how your system will behave under pressure.
Start out by creating your architecture being adaptable. Stay away from monolithic codebases where by anything is tightly connected. As an alternative, use modular structure or microservices. These patterns split your application into lesser, independent areas. Each individual module or provider can scale By itself without impacting The full procedure.
Also, consider your database from day just one. Will it need to manage one million users or simply a hundred? Pick the right sort—relational or NoSQL—based upon how your data will improve. Approach for sharding, indexing, and backups early, even if you don’t require them but.
A different vital stage is in order to avoid hardcoding assumptions. Don’t produce code that only works underneath present-day circumstances. Take into consideration what would transpire In the event your user base doubled tomorrow. Would your application crash? Would the database slow down?
Use style patterns that help scaling, like concept queues or celebration-pushed devices. These assistance your application tackle more requests with no having overloaded.
After you Establish with scalability in your mind, you are not just planning for achievement—you are lowering potential complications. A properly-planned technique is less complicated to keep up, adapt, and expand. It’s far better to prepare early than to rebuild afterwards.
Use the appropriate Database
Deciding on the appropriate database is really a key Element of making scalable programs. Not all databases are built a similar, and utilizing the Erroneous one can gradual you down as well as result in failures as your application grows.
Start off by comprehending your data. Could it be extremely structured, like rows inside of a desk? If Indeed, a relational database like PostgreSQL or MySQL is a great match. These are solid with associations, transactions, and regularity. Additionally they support scaling approaches like read through replicas, indexing, and partitioning to handle extra site visitors and data.
If the information is much more flexible—like consumer exercise logs, merchandise catalogs, or paperwork—take into consideration a NoSQL alternative like MongoDB, Cassandra, or DynamoDB. NoSQL databases are improved at dealing with substantial volumes of unstructured or semi-structured information and might scale horizontally much more quickly.
Also, think about your read through and publish patterns. Do you think you're doing a great deal of reads with much less writes? Use caching and read replicas. Do you think you're managing a hefty publish load? Take a look at databases which will handle large publish throughput, or simply event-primarily based knowledge storage units like Apache Kafka (for temporary info streams).
It’s also sensible to Assume forward. You may not will need Highly developed scaling attributes now, but selecting a database that supports them signifies you gained’t will need to modify afterwards.
Use indexing to speed up queries. Keep away from unwanted joins. Normalize or denormalize your details depending on your access patterns. And always check database functionality while you increase.
In short, the proper database depends upon your app’s structure, speed needs, And exactly how you hope it to mature. Choose time to select wisely—it’ll save a lot of trouble afterwards.
Improve Code and Queries
Rapid code is vital to scalability. As your app grows, each and every little delay provides up. Inadequately written code or unoptimized queries can decelerate effectiveness and overload your process. That’s why it’s essential to Create productive logic from the start.
Get started by producing clear, easy code. Steer clear of repeating logic and remove something unnecessary. Don’t pick the most sophisticated Answer if a straightforward one particular functions. Keep your features brief, concentrated, and simple to test. Use profiling tools to search out bottlenecks—areas exactly where your code can take also long to operate or utilizes far too much memory.
Following, take a look at your databases queries. These generally slow points down over the code alone. Make certain Each individual query only asks for the info you really have to have. Keep away from SELECT *, which fetches almost everything, and alternatively select distinct fields. Use indexes to hurry up lookups. And steer clear of executing too many joins, Specially throughout big tables.
In case you notice precisely the same details staying asked for repeatedly, use caching. Keep the effects temporarily employing applications like Redis or Memcached so that you don’t must repeat high priced functions.
Also, batch your database operations once you can. Rather than updating a row one by one, update them in groups. This cuts down on overhead and would make your application much more effective.
Remember to take a look at with significant datasets. Code and queries that work good with one hundred data could crash once they have to deal with 1 million.
In a nutshell, scalable applications are speedy applications. Keep the code limited, your queries lean, and use caching when needed. These actions assist your application remain easy and responsive, even as the load boosts.
Leverage Load Balancing and Caching
As your application grows, it's got to manage additional buyers and more traffic. If every thing goes via 1 server, it'll rapidly become a bottleneck. That’s where load balancing and caching are available in. These two tools help keep the application rapidly, steady, and scalable.
Load balancing spreads incoming site visitors across multiple servers. Instead of a person server accomplishing the many operate, the load balancer routes end users to unique servers determined by availability. This implies no single server receives overloaded. If one particular server goes down, the load balancer can deliver visitors to the Some others. Equipment like Nginx, HAProxy, or cloud-primarily based solutions from AWS and Google Cloud make this straightforward to put in place.
Caching is about storing info temporarily so it can be reused promptly. When consumers request the exact same data again—like an item webpage or a profile—you don’t should fetch it from your databases whenever. You are able to provide it from your cache.
There are two popular forms of caching:
1. Server-aspect caching (like Redis or Memcached) stores facts in memory for quick entry.
2. Customer-side caching (like browser caching or CDN caching) merchants static files near to the person.
Caching decreases database load, increases speed, and would make your app far more efficient.
Use caching for things that don’t improve usually. And normally ensure your cache is current when information does transform.
In short, load balancing and caching are basic but impressive instruments. Together, they help your application tackle a lot more people, stay quickly, and Get well from complications. If you plan to increase, you would like each.
Use Cloud and Container Equipment
To develop scalable purposes, you require applications that let your app expand quickly. That’s where by cloud platforms and containers come in. They give you versatility, lessen set up time, and make scaling Substantially smoother.
Cloud platforms like Amazon Web Solutions (AWS), Google Cloud Platform (GCP), and Microsoft Azure Allow you to lease servers and providers as you would like them. You don’t have to purchase hardware or guess long term capability. When site visitors will increase, it is possible to insert additional methods with just a couple clicks or mechanically working with car-scaling. When website traffic drops, you may scale down to economize.
These platforms also give services like managed databases, storage, load balancing, and security applications. You could deal with making your application as an alternative to controlling infrastructure.
Containers are Yet another important tool. A container offers your application and every little thing it must run—code, libraries, configurations—into one particular unit. This causes it to be simple to maneuver your application amongst environments, out of your laptop into the cloud, devoid of surprises. Docker is the most well-liked tool for this.
Once your app utilizes various containers, instruments like Kubernetes enable you to handle them. Kubernetes handles deployment, scaling, and Restoration. If a single part of one's application crashes, it restarts it instantly.
Containers also make it straightforward to independent aspects of your app into services. You may update or scale elements independently, which is perfect for performance and trustworthiness.
In brief, working with cloud and container resources usually means you'll be able to scale fast, deploy simply, and Get well quickly when troubles happen. If you need your application to expand without the need of limitations, start out using these equipment early. They help you save time, minimize possibility, and assist you to keep centered on building, not repairing.
Watch Every thing
For those who don’t keep track of your software, you received’t know when things go Improper. Checking can help the thing is how your app is executing, place challenges early, and make much better choices as your application grows. It’s a vital A part of creating scalable programs.
Get started by monitoring basic metrics like CPU usage, memory, disk Area, and reaction time. These show you how your servers and services are performing. Equipment like Prometheus, Grafana, Datadog, or New Relic can assist you accumulate and visualize this details.
Don’t just monitor your servers—monitor your app as well. Keep watch over how long it will take for consumers to load webpages, how often mistakes take place, and the place they come about. Logging instruments like ELK Stack (Elasticsearch, Logstash, Kibana) or Loggly may help you see what’s occurring within your code.
Arrange alerts for vital complications. Such as, if your reaction time goes earlier mentioned a Restrict or possibly a support goes down, you ought to get notified right away. This assists you repair issues speedy, normally in advance of end users even recognize.
Monitoring is also practical any time you make alterations. Should you deploy a whole new characteristic and find out a spike in glitches or slowdowns, it is possible to roll it back right before it will cause actual harm.
As your application grows, targeted traffic and info increase. Devoid of monitoring, you’ll pass up indications of difficulty right until it’s way too late. But with the proper instruments in position, you continue to be click here in control.
In short, checking helps you maintain your app reliable and scalable. It’s not almost recognizing failures—it’s about comprehension your system and making certain it works properly, even under pressure.
Ultimate Views
Scalability isn’t just for significant organizations. Even compact apps will need a strong Basis. By designing meticulously, optimizing sensibly, and using the suitable tools, it is possible to build apps that improve smoothly with no breaking under pressure. Start off small, Feel major, and Develop sensible.